Welcome to our comprehensive guide on Ruby hashes! In this tutorial, we’ll teach you everything you need to know about working with key-value pairs in Ruby. Whether you’re new to programming or a seasoned pro looking to expand your skills, this guide has something for everyone. We’ll start by covering the basics of creating and storing data in a Ruby hash, and then move on to more advanced topics like accessing and manipulating values, merging multiple hashes, and sorting data. Along the way, we’ll provide plenty of code examples and expert tips to help you master Ruby hashes.
In this guide, we will go over the following topics:
- Creating a Ruby Hash
- Storing Data in a Ruby Hash
- Accessing Values in a Ruby Hash
- Merging Two Ruby Hashes
- Using Multiple Values for a Single Key in a Ruby Hash
- Sorting a Ruby Hash
- Retrieving All Keys and Values from a Ruby Hash
- Summary
So let’s dive in and get started!
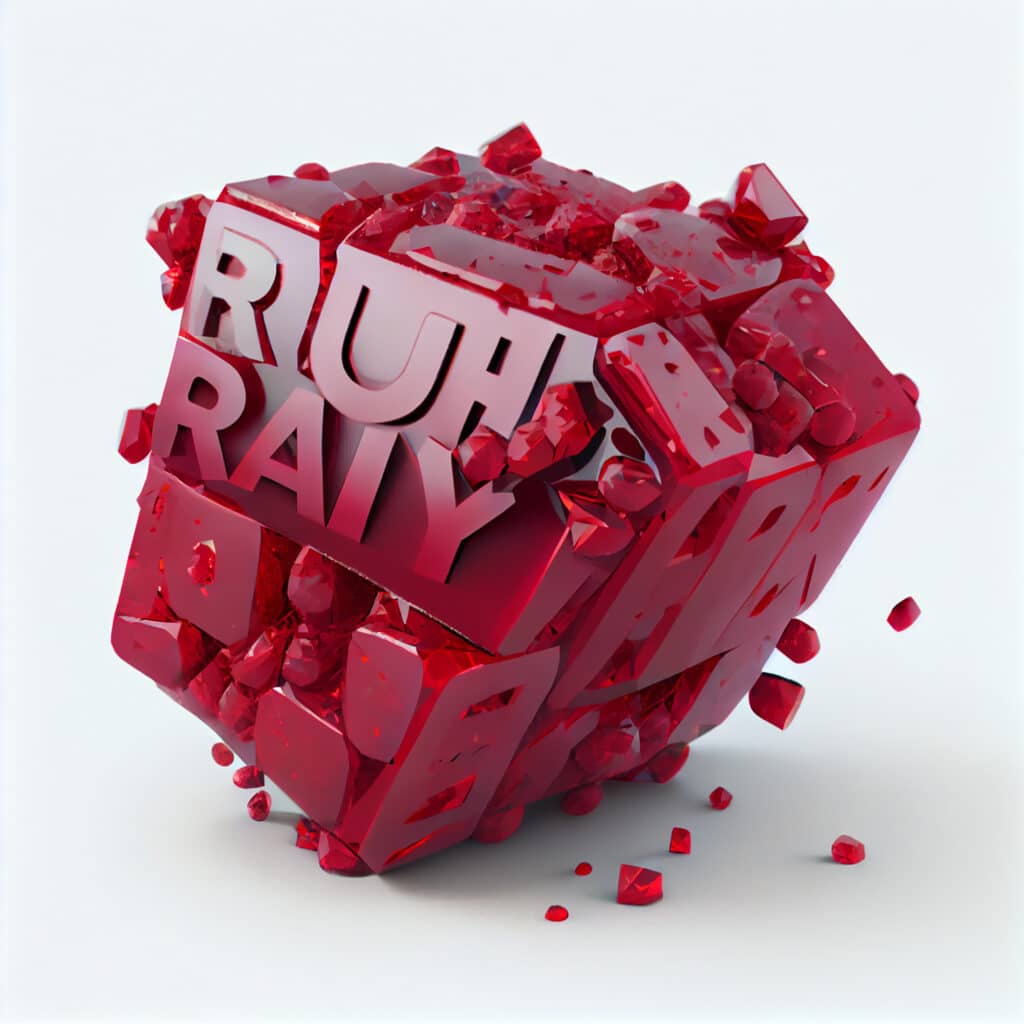
Creating a Ruby Hash
There are several ways to create a hash in Ruby. Here are a few examples:
# Method 1: Using the new method
h = Hash.new
# Method 2: Using the curly braces {}
h = {}
# Method 3: Using the hash rocket syntax (deprecated in Ruby 2.2)
h = { "a" => 1, "b" => 2 }
# Method 4: Using the symbol syntax (new in Ruby 2.2)
h = { a: 1, b: 2 }
Storing Data in a Ruby Hash
Once you have created a hash, you can store values in it by using the square brackets [] and the assignment operator (=).
# Create a new hash
h = {}
# Store a value in the hash
h[:key] = "value"
# Print the hash
puts h
# Output: { :key => "value" }
Accessing Values in a Ruby Hash
To retrieve a value from a hash, you can use the square brackets [] and the key of the value you want to retrieve.
# Create a new hash
h = { a: 1, b: 2 }
# Access a value from the hash
puts h[:a]
# Output: 1
If the key you are trying to access does not exist in the hash, you will get a nil value. You can also provide a default value that will be returned if the key does not exist in the hash:
# Create a new hash
h = { a: 1, b: 2 }
# Access a value from the hash that does not exist
puts h[:c]
# Output: nil
# Set a default value for keys that do not exist in the hash
h = Hash.new("default")
# Access a value from the hash that does not exist
puts h[:c]
# Output: "default"
Merging Two Ruby Hashes
You can merge two hashes together using the `merge` method:
# Create two hashes
h1 = { a: 1, b: 2 }
h2 = { c: 3, d: 4 }
# Merge the two hashes
h3 = h1.merge(h2)
# Print the merged hash
puts h3
# Output: { a: 1, b: 2, c: 3, d: 4 }
If both hashes have the same key, the value from the second hash will overwrite the value from the first hash:
# Create two hashes with the same key
h1 = { a: 1, b: 2 }
h2 = { a: 3, c: 4 }
# Merge the two hashes
h3 = h1.merge(h2)
# Print the merged hash
puts h3
# Output: { a: 3, b: 2, c: 4 }
Using Multiple Values for a Single Key in a Ruby Hash
In a Ruby hash, you can store multiple values for one key by using an array as the value:
# Create a new hash with an array as the value for one of the keys
h = { a: [1, 2, 3], b: 4 }
# Access the array from the hash
puts h[:a]
# Output: [1, 2, 3]
You can also store multiple values for one key by using a hash as the value:
# Create a new hash with a hash as the value for one of the keys
h = { a: { x: 1, y: 2 }, b: 4 }
# Access the inner hash from the outer hash
puts h[:a]
# Output: { x: 1, y: 2 }
Sorting a Ruby Hash
You can sort a Ruby hash by its keys or values using the sort method:
# Create a new hash
h = { a: 3, b: 2, c: 1 }
# Sort the hash by its keys
h1 = h.sort
puts h1
# Output: [ [:a, 3], [:b, 2], [:c, 1] ]
# Sort the hash by its values
h2 = h.sort { |a, b| a[1] <=> b[1] }
puts h2
# Output: [ [:c, 1], [:b, 2], [:a, 3] ]
Retrieving All Keys and Values from a Ruby Hash
To get all the keys from a hash, you can use the keys method:
# Create a new hash
h = { a: 1, b: 2, c: 3 }
# Get all the keys from the hash
keys = h.keys
puts keys
# Output: [:a, :b, :c]
To get all the values from a hash, you can use the values method:
# Create a new hash
h = { a: 1, b: 2, c: 3 }
# Get all the values from the hash
values = h.values
puts values
# Output: [1, 2, 3]
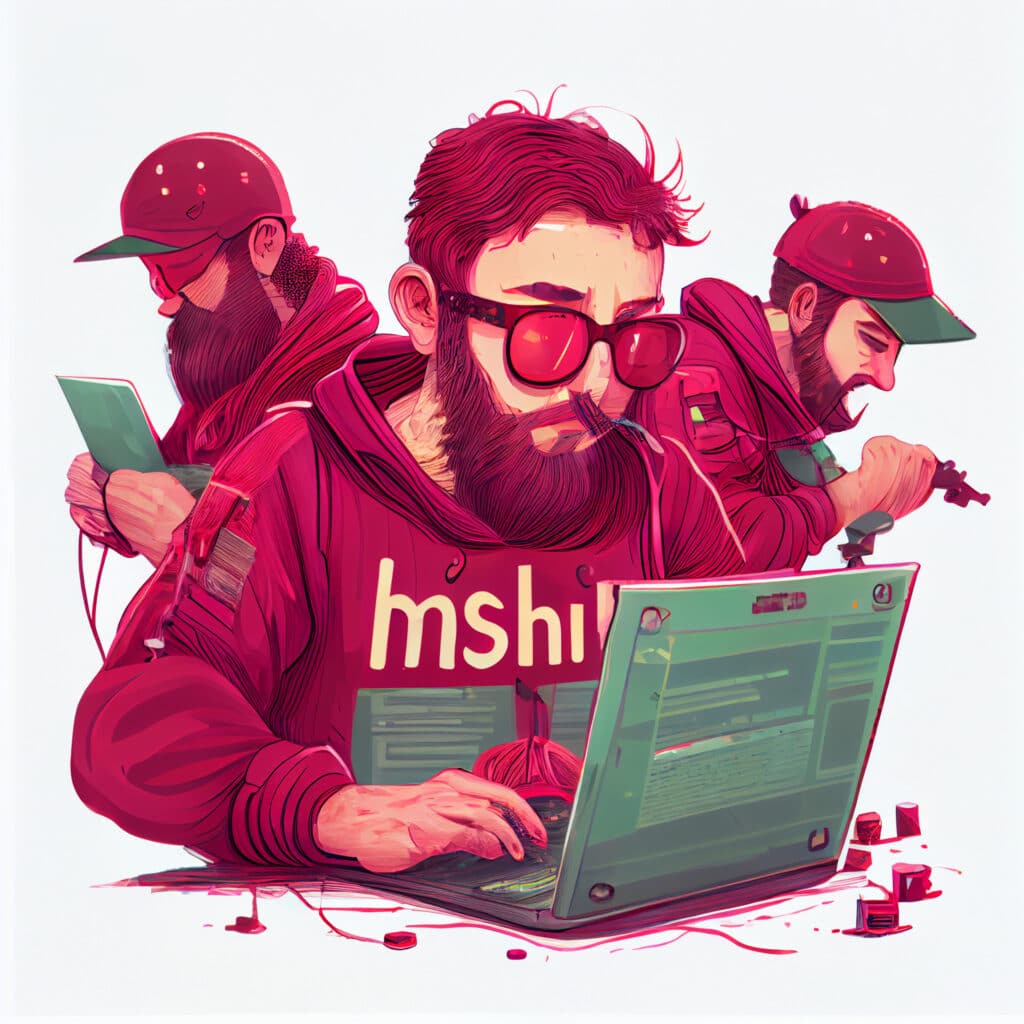
Summary
In this guide, we went over the basics of working with Ruby hashes. We learned how to create a hash, store values in a hash, access values from a hash, merge two hashes, store multiple values for one key, sort a hash, and get all the keys and values from a hash.
Hashes are a powerful and versatile data structure in Ruby that can be used to store and manipulate data in a variety of ways. With the knowledge you have gained from this guide, you should now have a good understanding of how to work with hashes in your Ruby programs.